The code for my processing twitter counter was pretty simple so I decided that I could have a go at building a fake version using js. I chose to make it fake because I did not want to get into any of the complications for needing to access the Twitter api as I'm not sure what they will be and would like to know I can get the underlying structure working first.
Again I made strong use of comments in this project so that I could easily refer to it and understand what was going on. For the tweets I just made a simple random number generating function which I used inplace of a real number of tweets.
/*current bugs
- suspected memory leak with currentTime function, animates even outside of the loop
-growth is not smooth. potentially something with how Im looping through my draw.
*/
//global variables
var countDuration = 10, //seconds. However js dose use millis.
globalCount = 0,
pGlobalCount = 0,
cp = 0,
time = 0, //this will return a value in seconds
context,
SCREEN_HEIGHT,
counterAmount = 10,
nodeDistance = 0,
nTweets = 0,
timeReset = 0,
s = 0,
//create builder
Counter = (function() {
//builder variables
var countDisplays = [],
canvas,
SCREEN_WIDTH;
//initiate
function init() {
measureScreen();
currentTime();
//sets up DOM elements
canvas = document.createElement('canvas');
context = canvas.getContext('2d');
setCanvasSize();
document.body.appendChild(canvas);
//loads counters elements
createDisplays();
//Starts Loop
update();
}
function measureScreen() {
SCREEN_WIDTH = window.innerWidth;
SCREEN_HEIGHT = window.innerHeight;
}
function setCanvasSize() {
canvas.width = SCREEN_WIDTH;
canvas.height = SCREEN_HEIGHT;
}
function clearContext() {
context.fillStyle = "rgb(0,0,0)";
context.fillRect(0,0,SCREEN_WIDTH,SCREEN_HEIGHT);
}
//Tweet facker
function fakeTweets(frequency) {
var random = (Math.random()*10)+1;
nTweets++;
if( nTweets === frequency) {
globalCount += random;
nTweets = 0;
}
}
//caluculates which counter should be counting
function countPosition() {
if (currentTime() - timeReset === countDuration) {
//if (currentTime() % countDuration === 0) { //THIS IS BUGGED STICKS ON 4
timeReset = currentTime();
cp++;
pGlobalCount = globalCount;
}
if (cp === counterAmount) cp = 0;
}
//recursive function which increase time by 1 every second.
function currentTime() {
time++;
return (time/60).toFixed(0); //toFixed lets you round to X decimal places. in this case 0.
//setTimeout(startTime,1000);
}
///////////////////////////////////////////////////////
// COUNTERS
//renders the counters
function drawCountDisplays() {
//runs through all the counters in the array
for(i = 0; i < countDisplays.length; i++) {
var current = countDisplays[i];
// checks if the current array should be counting
if(i === cp) {
current.counting();
current.size = current.counting();
current.countRender();
} else {
current.countRender();
}
}
}
//populates an array with count displayers
function createDisplays() {
for(i = 0; i < counterAmount; i++ ) {
//xpos: {SCREEN_WIDTH / (counterAmount - i)};
//xpos++;
nodeDistance = SCREEN_WIDTH / counterAmount;
countDisplays.push(
new CountDisplay((i+0.5) * nodeDistance, 0));
}
}
// end of counters
///////////////////////////////////////////////////////
//DEBUG PRINTER
function debug() {
document.getElementById("globalcount").innerHTML= "Global Count = " + globalCount;
document.getElementById("pglobalcount").innerHTML= "Previous Global Count = " + pGlobalCount;
document.getElementById("countposition").innerHTML= "Counter Position = " + cp;
document.getElementById("timereset").innerHTML= "Time reset = " + timeReset + "sec";
document.getElementById("time").innerHTML= "Time = " + currentTime() + "sec";
}
function render() {
currentTime();
fakeTweets(25);
//createDisplays();
countPosition();
drawCountDisplays();
debug();
}
//the loop - I think This is a recursive function.
function update() {
clearContext();
requestAnimFrame(update);
render();
//fakeTweets(25);
//countPosition();
//drawCountDisplays();
}
//api
return {
init: init
};
})();
// Constructer for Count Display Objects
function CountDisplay(xpos, size) {
this.size = size;
count = 0;
//counts the amount of tweets
this.counting = function() {
count = globalCount-pGlobalCount;
return count;
}
//draws the counter based on the amount of tweets
this.countRender = function(){
context.fillStyle = 'rgba(255,255,255,0.5)';
context.beginPath();
context.arc(xpos, SCREEN_HEIGHT/2, this.size, 0, Math.PI * 2, true);
//context.arc(xpos, 200, 50, 0, Math.PI * 2, true);
context.closePath();
context.fill();
}
};
//starts code
window.onload = function() {
Counter.init();
};
Its steadily getting more and more complicated! and I've not even started on the web sever. This took a little more work as I had a few simple bugs which due to how new I am to JS I couldn't spot. There are still some bugs in this any way, as you can see from the top line. I have not put this online but here is a screen grab of it in action
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I've never done any JS before for I decided a good way to start was to do some of the examples from the website Codecademy so that I could see how the structure of things such as arrays and variables where in JS. In total I did a little over 100 exercises on the site covering the basics and object oriented programming.
Alongside this I also downloaded some example projects from CreativeJS by Paul Lewis and Seb Lee-Delisle. I thought it would be a good idea to look at Seb's coding as he taught me everything I know about programming so far so it might have similarities in the structure and approach to it. I looked thought these projects and tried to look for the various section that appear in processing such as the draw loop and the setup, by doing this I was able to understand the structure of JS. Through doing this I was introduced to some new concepts such as DOM, Document Object Model, and a better understanding of API, application programming interface.
I wanted to start off really simple, so instead of building twitter counter straight away I build a simple bouncing ball in js. I made sure that I hand wrote everything so that I would be able to spot when I made mistakes and would actually be learning. I also made sure to comment my code so that I would understand what every section was doing.
//load up my bounce class
var Bounce = (function() {
var canvas = null,
context = null,
SCREEN_WIDTH = 0,
SCREEN_HEIGHT = 0,
//ball variables
xPos = 500,
yPos = 500,
xVel = 10,
yVel = 9,
radi = 50;
// init the code
function init() {
measureScreen();
//creates DOM elements
canvas = document.createElement('canvas');
context = canvas.getContext('2d');
setCanvasSize();
document.body.appendChild(canvas);
//StartsLoop
update();
}
function measureScreen() {
SCREEN_WIDTH = window.innerWidth;
SCREEN_HEIGHT = window.innerHeight;
}
function setCanvasSize() {
canvas.width = SCREEN_WIDTH;
canvas.height = SCREEN_HEIGHT;
}
function clearContext() {
context.fillStyle = "rgba(255,255,255,1)";
context.fillRect(0, 0, SCREEN_WIDTH, SCREEN_HEIGHT);
}
// draws bouncing ball
function draw() {
xPos += xVel;
yPos += yVel;
// x cordinate bounce
if (xPos > SCREEN_WIDTH - radi) {
xVel *= -1;
xPos = SCREEN_WIDTH - radi;
}
if (xPos < radi) {
xVel *= -1;
xPos = radi;
}
// y cordinate bounce
if (yPos > SCREEN_HEIGHT - radi) {
yVel *= -1;
yPos = SCREEN_HEIGHT - radi;
}
if (yPos < radi) {
yVel *= -1;
yPos = radi;
}
context.fillStyle = 'rgb(200,255,200)';
context.beginPath();
context.arc( xPos, yPos, radi*2, 0, Math.PI * 2, true );
context.closePath();
context.fill();
}
//Loop
function update() {
clearContext();
requestAnimFrame(update);
draw();
}
//api
return {init: init};
})();
//start my code when window opens
window.onload = function() {
Bounce.init();
};
I have put a live version on my site. As you can see its a little buggy but I'm pretty happy with how it turned out and learn a lot writing it. The project runs in html5 using the jS to put the canvas element in the html when the page loads, this is so it will be full screen regardless of screen size.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I was quite keen with this project to use it to enable me to learn JavaScript. It had been my intention to recreate my processing project in JavaScript before I found out about the repeat api use issue. Now however I have found out about Node.js which is defined on their site as,
Node.js is a platform built on Chrome's JavaScript runtime for easily building fast, scalable network applications. Node.js uses an event-driven, non-blocking I/O model that makes it lightweight and efficient, perfect for data-intensive real-time applications that run across distributed devices.
This is perfect for what I want to do as it will all be in JS so that I can spend more time learning it and it will enable me to have my api section running server side.
Node.js looks really advanced however so I'm going to start by learning some JS and then porting my existing processing prototype to JS.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

After doing some more reading about the Twitter streaming api I came across a paragraph which I had not noticed before.
Each account may create only one standing connection to the Streaming API. Subsequent connections from the same account may cause previously established connections to be disconnected. Excessive connection attempts, regardless of success, will result in an automatic ban of the client's IP address. Continually failing connections will result in your IP address being blacklisted from all Twitter access.
The impact of this means that I can not have each user run a version of my code otherwise I would be running multiple connections and would risk an automatic ban. There is the possibility of using a 'site stream' though you have to apply for this and I do not want to waste time waiting to see if I'll get one.
The way around this is to have my code running on a websever which is always active which then users request the information from when they visit the site. I like this as it lets me follow my idea of the site as an object. However I've no idea about server side programming so I need to do some research.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

Currently I know that I want my visualisation to be delivered online but I'm not sure what I'm going to build it with. However to understand the processes involved I've decided to mess around with processing and the Twitter api.
There is a Java Libary which I found on a blog post by Jer Thorpe called Twitter4j which will let me access the twitter api. I created my api key by going to the developer section of twitter then messed around with some code I found so that I could understand what each of the sections did.
The first project uses the twitter search api. It was built by going through this Jer Thorpe tutorial then modding the code slightly.
This project searches a given time period for a random sample of X tweets containing Y, in this example X is 10 and Y is "Sleep".
The search api is good but it only gives you access to existing tweets not ones which are coming in live for this I need to use the streaming api.
I found an example by Michael Zick Doherty of using Twitter4j to access the streaming api and display images when they come in on a chosen subject. By looking at this and decompiling the Twitter4j library so I could understand its structure and how the classes worked.
With this knowledge I was able to write a prototype that I could increment a variable when ever a tweet came in. Once I had this I was able to create a display which would show this amount over time. One thing which was really useful while building it was to create a little debug panel which I could look at the amounts in my current variables and spot if there where any errors.
//Search term
String keywords[] = {"breakfast"};
//Global variables
int countDuration = 60000; //how long before the counter moves on (in miliseconds)
int globalCount;
int pGlobalCount; //previous global count value
int cp = 0; //counter position
float time = 0;
TweetCount[] tweetCount = new TweetCount[59]; //amount of bits of data to collect
TwitterStream twitter = new TwitterStreamFactory().getInstance();
// Twitter API variables
static String OAuthConsumerKey = "XXXXXXXXXXXXX";
static String OAuthConsumerSecret = "XXXXXXXXXXXX";
static String AccessToken = "XXXXXXXXXXXXXX";
static String AccessTokenSecret = "XXXXXXXXXXXXXXXXXXXXX";
//////////////////
//////////////////
void setup() {
size(1000, 400);
noStroke();
imageMode(CENTER);
smooth();
connectTwitter();
twitter.addListener(listener);
twitter.filter(new FilterQuery().track(keywords)); // This is where my filters come into the code
for(int i=0; itweetCount[i] = new TweetCount();
}
}
void draw() {
background(0);
debug();
CountPosition();
for(int i=0; ifloat xpos = map(i, 0, tweetCount.length, 0, width);
tweetCount[i].render(xpos);
}
tweetCount[cp].counting();
}
// Initial connection
void connectTwitter() {
twitter.setOAuthConsumer(OAuthConsumerKey, OAuthConsumerSecret);
AccessToken accessToken = loadAccessToken();
twitter.setOAuthAccessToken(accessToken);
}
// Loading up the access token
private static AccessToken loadAccessToken() {
return new AccessToken(AccessToken, AccessTokenSecret);
}
// This listens for new tweet
StatusListener listener = new StatusListener(){
public void onStatus(Status status) {
//println("@" + status.getUser().getScreenName() + " - " + status.getText());
globalCount++;
}
public void onDeletionNotice(StatusDeletionNotice statusDeletionNotice) {
//System.out.println("Got a status deletion notice id:" + statusDeletionNotice.getStatusId());
}
public void onTrackLimitationNotice(int numberOfLimitedStatuses) {
// System.out.println("Got track limitation notice:" + numberOfLimitedStatuses);
}
public void onScrubGeo(long userId, long upToStatusId) {
System.out.println("Got scrub_geo event userId:" + userId + " upToStatusId:" + upToStatusId);
}
public void onException(Exception ex) {
ex.printStackTrace();
}
};
void CountPosition() {
if(millis()-time >= countDuration) {
time = millis();
cp++;
pGlobalCount = globalCount;
}
if (cp==tweetCount.length) cp = 0; //counter postion reset
}
void debug() {
text("globalCount =" + globalCount, 20, 20);
text("pGlobalCount =" + pGlobalCount, 200, 20);
text("CountPosition =" + cp, 20, 40);
text("time =" + millis()/1000, 20, 60);
}
class TweetCount {
int count;
int countreset;
TweetCount() {
}
void render(float xpos) {
fill(255,100);
ellipse(xpos, height/2,count,count);
fill(255);
text(count, xpos, height/2+70);
}
void counting() {
count = 0;
count = globalCount-pGlobalCount;
//println("count =" + count + " globalCount =" + globalCount + " pGlobalCount =" + pGlobalCount);
}
}
At this stage I am happy with my knowledge of how the code side of it works I just need to look into how I will make this available online
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I've now settled on a new idea. I got a little lost thinking about interesting ways in which I could use images from Instgram. I did want to create a site which would show images of what people are eating in real time based on the images coming in from Instgram (I may still try build this sometime as it sounds like a fun project). Now though I've decided that I'm just going to draw my data from twitter.
The basic idea is that I want to create an abstracted real time clock based on people talking about lateness on twitter. Hopefully this will produce an interesting clash of ideas. I am still keen on it being created for a gallery space but I also want it to be accessible online so that, like the data its based on, it's not spatially limited.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

Glitchhiker was the winner of the 2011 Global Game Jam NL. Its a simple game with a fantastic mechanic, the game can die. Infact it is already dead. The game works by having a global life count, starting the game uses a life and collecting 100 coins gains a life. Once the lifes reach 0 the game is no longer playable or downloadable so it dies!
This is quite a similar concept to what I picked up from Iain M banks. I think it would be really interesting to build a world from data which only develops based on if people are using it. I can picture this in a few forms;
- A virtual world which grows over time as it is viewed at a speed based on the amount of people viewing it. If no one or a limited number of users are viewing it then it will degenerate with time until its being watched again.
- A generative narrative animation which progresses when ever someone is viewing it so that if you come back to it you will miss parts of it. This would work really well if it was a 24 hour loop so it would encourage people who are interested in the story to visit regularly so that they can know where they are within the story and then catch up. This would need a story which did not depend on traditional narrative structures, such as the Golden Notebook by Dorris Lessing
This kind of approach also in this idea I was talking about previously in my blog about treating the website like a real thing which has a life away from the user. This contrasts with the classic view of it being a portal to dispense information.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

Well I have written a few notes in my sketch book based on a new idea of displaying some form of life data online. As they are in my personal sketchbook like before I'm going to upload them here as I will still need to use my sketchbook! I also played around with some different ideas here as well.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

After talking to my tutors about my idea they did not think that I would be able to get enough data to realise my idea in a worthwhile way. They suggested that I use my strong interest in data and switch to using a data set which I do not have to create myself such as Twitter or Instagram to base my idea on. They also think that I should draw on some of the ideas from my dissertation, which talks about lifelogging and the increase of data about a users life which is being gathered and made available online.
I was quite excited about building this visualisation but I do agree with my tutors about this idea not being something which I have the resources to create at the moment. So now I've got to think of a new idea!
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

As I would like to build some form of device to feed data to my visualisation I have order myself an Arduino Uno. But until that arrieves I throught it would be a good idea to see if I could build a quick prototype in processing which would graphically display the volume of a mic input. I decided to ignore the web limitations for now as I had no idea how easy it would be to put together.
I used Minium, a sound library for processing. Which I discovered records the volume from the left and right channel with a value between 0 and 1. This meant that with a very small amount of code I was able to create a simple visualisation which changed the colour of a circle based on the volume.
// load libaries
import ddf.minim.*;
// minium vars
Minim minim;
AudioInput input;
void setup(){
size(500,500);
smooth();
noStroke();
colorMode(HSB);
minim = new Minim(this);
input = minim.getLineIn();
}
void draw() {
background(0);
fill(volume(),255,255);
ellipse(width/2,height/2,100,100);
println(input);
}
float volume() {
return(input.mix.get(1)*1000);
}
void stop(){
input.close();
minim.stop();
super.stop();
}
even with something simple like this if you where to have a large amount of them positioned relative to there location you would get a really interesting image
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

When looking for inspiration on the potential forms which my sound data could be displayed in I came across these abstracted literal tube maps at Line Posters.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I found an example of a great little web based visualisation. Color Forecast shows what people are wearing in key fashion capitals around the world in real time. Its has been made by an online clothes retailer and will suggest what clothes you should wear to go with the city. It also shows a little bit of the history of the data and shows its process.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I was not under the impression that my idea was completely new but I was not aware of any examples. That was until I read a line In Emotional Geographies, a book I've been reading because I think I'm aiming to visualise an emotional geography of the noise of life. The line was about the desire to "decouple diological and military concers" which made me think of a machine they build in The Dark knight which make a large sonar real time map of the city from the sound provided through mobile devices... pretty much a literal visual version of what I wanted to build. Incase you have not seen the film here is a clip
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

When I was imagining how a visualisation of location mapped sound might look the work of Aaron Koblin came to mind. In particular one project which he creates a visualisation tool for SMS messages in Amsterdam,
The video really shows the patterns which appear in this style of data. It looks almost like bar charts but they are mapped in 3d space. I would like to create a 3D space within the website which could be explored by the user so that they can focus on the section of the data they are personally interested in
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

At this point I thought it would be a good idea to review my idea and try and finally settle on a project which I can start creating. So I discussed the ideas through with my mum, Sarah Scaife. We go to the point that pushing on with the idea of data was my best bet as I already have some of the skills needed to do this and would not be starting from scratch like I might where I to build a physical object manipulated by the internet.
Like how the hint.fm wind map using pubicaly available data I thought it would be a good idea if I used data which as already accessible as that brings its own potential interpretations to the piece. So I had a look on the Uk open goverment data. However I looking at what was available nothing jumped out at me as being a really interesting direction to go with.
When Thinking of the variables available to me I got quite interested in sound. So at this point I decided that I would like to create something similar to the wind map but using sound data from specially build devices. The idea being that with ubiquity of devices which record sound you would be able to tell a large amount of things from this data. One example would be that you could find out how busy a cue for a club was from the amount of volume coming from outside it.
I really like this Idea and I'm going to go with it. I think that with clever application of what I'm displaying and recording this could produce a nice piece.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I am still interested in the uses of data so decided to look more into data visualisation to see how those ideas could spread through into my work. While doing this I came across a visualisation of the wind in the USA by Hint.fm. I This this is a great idea and is really nicely executed.
I can really imagine the map being displayed in a gallery space. It scrapes its information every 30mins but stays dynamic and engaging with this way in which it displays its data. They also show information about visualisation alongside it which I think works well when seen over a website but if this were in a gallery I would like to only see the map.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I have had quite a few idea on different things which I would like to produce so far which you can see in the pages on my sketch books. They mainly revolve around creating a digitally controlled object. My ideas so far are:
- To create a gallery piece where two crt monitors displaying faces are set facing each other. People would be able to visit a website and take control of one of the monitors in order to have a conversation with people using the other monitor. The aim would be to create a way in which people can engage in "real" conversation digitally. It would try to explore ideas around privacy and our concepts on communicating over time. I could sum it up really basically by saying its annomous skyping with random people while others watch in a real location.
- To hack a type writer so that it would create a physical copy of digital tweets.
- To create a multiplayer web game where the course which the players act within is controlled by a physical object presented in a public or gallery space. A quick way in which I though of this working was to make a version of Astroids where instead of destroying Astroids the players work together to destroy objects which people using the physical gameworld controls are creating.
- As above but instead of generating a game it would create some form of dynamic art piece which could be both projected within the space and viewed online.
- I also thought of reversing the roles between online and physical space by creating some form of projection which online players could attack and distort, therefore having a direct impact on the work on display.
With this ideas I am quite worried that after looking at some Arduino tutorials and thinking about how I could go about creating them that I might be very technically challenging.
I spoke with one of my tutors about the first Idea and he was interested in the direction it was going but felt like it could be pushed even further. He suggested that I could play on the idea of control and dominance by altering the size and positions of the displays so to users it feels like one of them is "stronger".
Alot of these ideas have a common theme of a tangible web based element which is the same to all who are viewing it. I think this is really interesting and I plan to explore this idea more.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I want to look at this project from a nostalgic sci-fi perspective. With that in mind I thought a good way to build up more of an opinion on my perceptions of this would be to read some old sci-fi literature. I got 3 books from my local 2nd hand book store,
- Ender's Game by Orson Scott Card
- Burning Chrome by William Gibson
- The State of the Art by Iain M. Banks
Burning Chrome
This was the first book I got. I chose it because I have previously read Neuromancer and it had just the sort of feel I was going for. Also as Gibson invented the word cyberspace I thought he was bound to have the sort of ideas I was thinking of.
The book is a collection of short stories covering a wide range of topics. They have strong cowboy like male characters and the worlds come across as being really rough. There are a lot of dark themes throughout the stories and you can see that I wrote in my sketch book DOUBT in big letters and this was a recurring emotion I thought was coming from the characters.
As for the Technology It was all pretty advanced. There where some interesting descriptions about the UX of the users,
"A silver tide of phosphenes boiled across my field of vision as the matrix began to unfold in my head, a 3-D chessboard, infinite and perfectly transparent. The Russian program seemed to lurch as we entered the grid."
The description all seem really physical and as if you could feel them using words such as 'boiled' to explain movement. He also uses the acronym ICE which brings more feelings to mind when you imagine it. By talking about the physical effects on the users, such as sweating, it really sounds like an intense experience. While it would be very hard brining in this sense of something digital being felt and having an impact on your real world experience would be a really nice quality to build into my project.
Enders Game
I got Enders Game as it was a book which I had hear good things about but never read. I didn't really know what to expect but I ended up really enjoying it. *Spoliers* It's basically an account of how a future society groom a young boy to be able to wipe out a whole race of intelligent aliens. Parts of the book talk about Enders siblings who use the anonymity of the internet to influence political ideas of peoples and nations.
The technology was not a massive part of this book. Though I may think this because the visions of the technology are slightly more extreme versions of devices we have now. One of the main pieces if a 'Desk' which is essentially a large iPad with an app on it which is based on the users brain signal. I quite like its method of punishing the user for failing tasks. On each progressive fail the game sets you further back within the level (its more like a continuos world). This would be really irritating to play and think it would be interesting making a very difficult game designed to frustrate the user which makes use of this.
The State of the Art
This was defiantly the most surreal of the books I read, with stories such as an astronaut landing on a planet which has intelligent plant based lifeforms who then pluck his limbs off! It is another collection of short stories. At first I didn't like them as they have odd amounts of non human lifeforms in them, and some have weird structures.
The stories point out alot of pointless self destruction. Which I think is quite an interesting thing to highlight it would be nice if my project could deliberately damage itself. Again I don't think the technology is key in these stories, its about the conscious effect of the power they bring and the relationship which build up between the user and the device. These are powerful themes when it comes to trying to explore new areas within digital design but they do no themselves suggest a device or object to be created.
From reading these books I've learn't that whats going to make my project work is keeping it grounded in human experience and emotions. Doing so will allow people to empathise with what I create.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I have been using my personal note book as a sketch book for this project so far so I decided that I would upload the notes and ideas I have had so far.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I've found some interesting methods of repetitive drawing. I was drawn to these by there mechanical process and the idea that this is something which could make use of an Arduino. Found on Brain pickings
I Think it would be really interesting to produce something physical in real time from digital information and building a device or adopting mechanical processes would allow me to explore this.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

Makego is an interesting iPhone app which is a step on the way towards blurring the boundaries between physical and digital play.
While I don't think this takes it very far It is an interesting idea and one which I know I would have loved when I was a kid. I also think its quite significant that something which is design for potential rough play from younger kids has been make for iPhone an expensive piece of kit which potential has a large impact on its owner if its broken. I also like that it creates yet another potential use for a mobile far beyond the original ability to talk on the phone while away from a landline.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

As I've already laid out I'm interested in looking at digital technologies from a sci-fi perspective, so I thought I may as well listen to sci-fi music while I work as well. So I've been listening to the Bladerunner soundtrack online, Its pretty good! If my piece ends up using audio I can draw inspiration from this as it really communicates the scenes and emotions both in the film and within sci-fi literature.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

While looking for interesting angles I can take on this project I have still been keeping an eye on interesting new technologies. One which really excited me was a bbc article explaining using conductive ink to produce paper posters which can play music!
While this sort of technology is out of my reach in terms of something which I could produce for this project I really like the concept of combining traditional means of communication in a way which either doesn't alter the appearance of the one of original projects or takes it into a new object.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

I first heard of Hellicar & Lewis in the elevator pitch at flash on the beach. They make really interesting interactive digital pieces. There work gets displayed in galleries and shows good foundations for the future possibilities of digital art. One of there pieces The Hello Wall was a large outdoor projection which should change in response to users tweets.
The piece is very simplified in this form but due to the user interaction has an endless amount of outcomes. They did develop the concept further with The Hello Cube which was at the Tate Modern at the end of march.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

A few years ago I went to OFFF in paris. The theme for that years conference was "Nostalgia for a Past Future" which I though was a really interesting idea. By looking at this project from a sci-fi prospective I hope to bring is a feeling of this past vision of the future. Recently The Guardian posted an image which sums up some of utopian thinking which used to happen in reguards to the future.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

Some one was tweeting about the flash game You Have to Burn the Rope. I played it through and its really great I suggest you go and play it now before you carry on reading.
The game is more of a comment about current flash game development than a game. Its a bout the process of playing to the end of something with a majority of the enjoyment coming from the ending credits of the game. The game takes 3 mins and only one of those is gameplay.
I felt this was relevant to post as it manages to communicate its message and stay engaging while still being very short. I want my piece to be able to communicate its purpose very quickly.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

Niklas Roy is a digital artist I came across who makes a wide range of strange digital objects. His work play with a mix of traditional technologies and futuristic ideas. One of my favorite works by him is the Electronic Instant Camera.
My ‘Electronic Instant Camera’, is a combination of an analog b/w videocamera and a thermal receipt printer. The device is something in between a Polaroid camera and a digital camera. The camera doesn’t store the pictures on film or digital medium, but prints a photo directly on a roll of cheap receipt paper while it is taking it. As this all happens very slow, people have to stay still for about three minutes until a full portrait photo is taken.
This piece reminds me lots of a modernday version of a dry plate camera. I really like how it takes a long time to generate the image and its not stored so that unlike how I would use a camera with my friends, by taking a load of photos and picking the best, you have to think much more about the composition and just be content with the image if there are imperfections.
The way it builds up in lines also makes me think about the possibilities for collaborating and having multiple people each take one line each like the drawing game children play.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

While looking for more information about what is possible with an Arduino I found this series of tutorials by electrical engineering major Jeremy Blum, which has 5 hours worth of videos!
This first video of the series is a good introduction and he links to some of the projects he has made which has some really exciting examples. The interesting thing about the linked projects being Arduino based is that they will always produce a real object.
one of his examples he linked up web data to a LED which it self is not that impressive but it has the same principle of the remote control cafe. I could for example make some moving sculpture which changes state bases on online information.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

A few months Kauko came up in my rss reader and it was what sparked my interest with the ability for digital technologies to have a direct impact on real world spaces.
One of the things that stuck out about this is that you could move objects through the internet. It changed the concept of the internet as being for consumption as to something which could be used for direct physical action. While it was active I had a go with it and it feels really funny being able to see live reactions to your actions, knowing that you are completely anonymous to those involved.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

The Raspberry Pi has recently been released. It is a working computer which can run linux, is very very small, and very cheap. This is a very popular piece of equipment and with only a limited number available and demand spiking at 700per second it may be quite hard to get my hands on one.
This piece of technology is really exciting as it would allow me to have computational power within an object. It is able to run of battery power so using this I would be able to have a wireless object which could communicate with a web based service.
Another micro computer is the Arduino. This is not a fully working computer but a device which lets you have real world inputs and outputs.
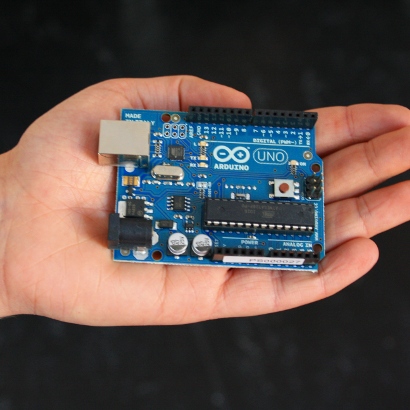
Connecting an Arduino with a raspberry pi quickly opens up a large amount of possibilities for creating interactive objects. You can control an Arduino with processing which has a good community for support. I also found Element 14 which is a community of people interested in computer science. I am anxious that building an interactive object of this sort requires quite a few skills which I do not have so it seems quite ambitious.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit

As part of our project we are required to create a document outlining out intentions on what we will produce, its pretty much a brief.
I have intentionally kept my brief really open. I'm interested in the ideas and processes of digital technology and want to explore the possibilities some more before I decide exactly what I will produce. That said I have limited myself to producing an object. I want to look at the boundaries between a physical and digital reality. This is potentially a bad idea as when I'm doing research and thinking of idea I already have a desired outcome so they have to be able to fit that.
- Leave your comment • Category: Project 5: Independent Project
- Share on Twitter, Facebook, Delicious, Digg, Reddit
